You can create multi-part numerical questions that require your students to enter their observed or estimated data and to perform calculations based on their data. These questions are often used for lab classes to record the results of an experiment and perform analysis of the data.
- Prerequisites
- Create Fraction Questions
- Allows students to enter observed or estimated numerical data. Any response that falls within a defined range of values is scored correct.
- Optionally, provides feedback to students for observed or estimated numerical data based on whether the value is lower than, higher than, or within the range of acceptable values.
- Requires students to perform calculations based on the provided data points.
- Enforces sequential entry of data and calculations so students cannot enter the results of calculations before entering the required data. The answer boxes for calculations are not enabled until your students enter the data required for the calculation.
- Indicates to students when a calculation was performed correctly based on underlying data that are not within the range of acceptable values. Each calculation is scored correct only if the calculation was performed correctly and the underlying data are within the range of acceptable values.
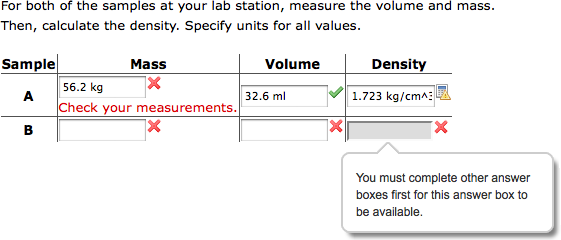
Like all numerical questions, answer-dependent questions can require students to specify units or to use a specified number of significant digits or decimal places in their responses.
Example Answer-Dependent Question Without Feedback
The following table summarizes an actual question.
QID |
|
---|---|
Name |
|
Mode |
|
Question |
|
Answer |
|
Display to Students |
![]() |
Example Answer-Dependent Question With Feedback and Units
The following table summarizes an actual question.
QID |
|
---|---|
Name |
|
Mode |
|
Question |
|
Answer |
|
Display to Students |
![]() |